Research System Setup (Observer Pattern)
Programming Pattern: Observer Pattern
UI Setup
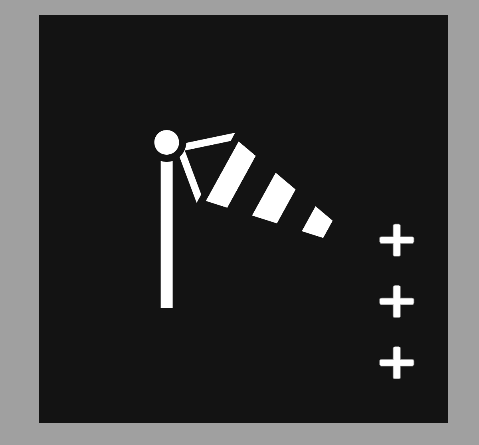
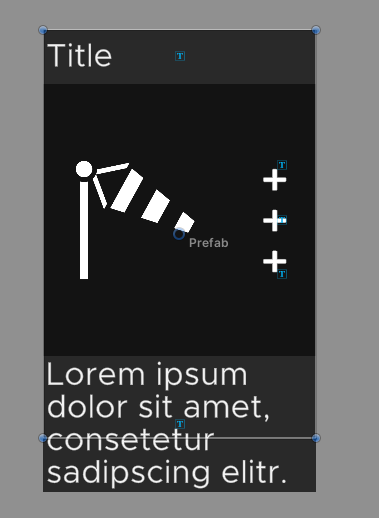
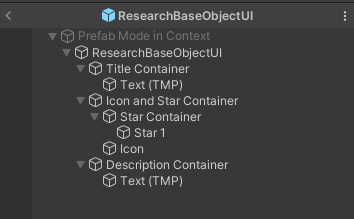
The ResearchBaseObjectUIData
class is a data container that holds UI-related references. (like TextMeshPro Component, or the Icon Image Component)
using UnityEngine;
public class ResearchBaseObjectUI : MonoBehaviour
{
public ResearchBaseObjectUIData UIData;
public void Assign(ResearchBaseSO so)
{
if (!UIData.IsEmpty())
{
UIData.IconImage.sprite = so.Icon;
UIData.Title.text = so.Name;
UIData.Description.text = so.Description;
UIData.Stars.text = "";
}
so.OnResearchCompleted += Complete;
}
private void Complete()
{
UIData.Stars.text += "+";
}
}
Let’s generate research objects.
At the moment there are only UI assigments. ResearchBaseSO
contains research data.
Our Research Controller contains a list of ResearchBaseSO
.
Lets spawn an object out of that list. Then store it somewhere. To access the spawned object, store it inside the ResearchController.
ResearchController contains a reference to the Base UI Prefab to instantiate and to assign scriptable objects to each instance:
using System.Collections.Generic;
using UnityEngine;
public class ResearchController : MonoBehaviour
{
public List<ResearchBaseSO> researchList;
[SerializeField] private GameObject researchPrefab;
private void Start()
{
foreach (ResearchBaseSO research in researchList)
{
Instantiate(researchPrefab);
researchPrefab.GetComponent<ResearchBaseObjectUI>().Assign(research);
}
}
}
Also a list to store spawned objects is required, and a container to display ui objects properly:
using System.Collections.Generic;
using UnityEngine;
public class ResearchController : MonoBehaviour
{
public List<ResearchBaseSO> researchList;
[SerializeField] private GameObject researchPrefab;
[SerializeField] private Transform container;
private List<ResearchBaseObjectUI> storedResearchObjects = new List<ResearchBaseObjectUI>();
private void Start()
{
foreach (ResearchBaseSO research in researchList)
{
ResearchBaseObjectUI instance = Instantiate(researchPrefab, container).GetComponent<ResearchBaseObjectUI>();
instance.Assign(research);
storedResearchObjects.Add(instance);
}
}
}
voilá – first research item:
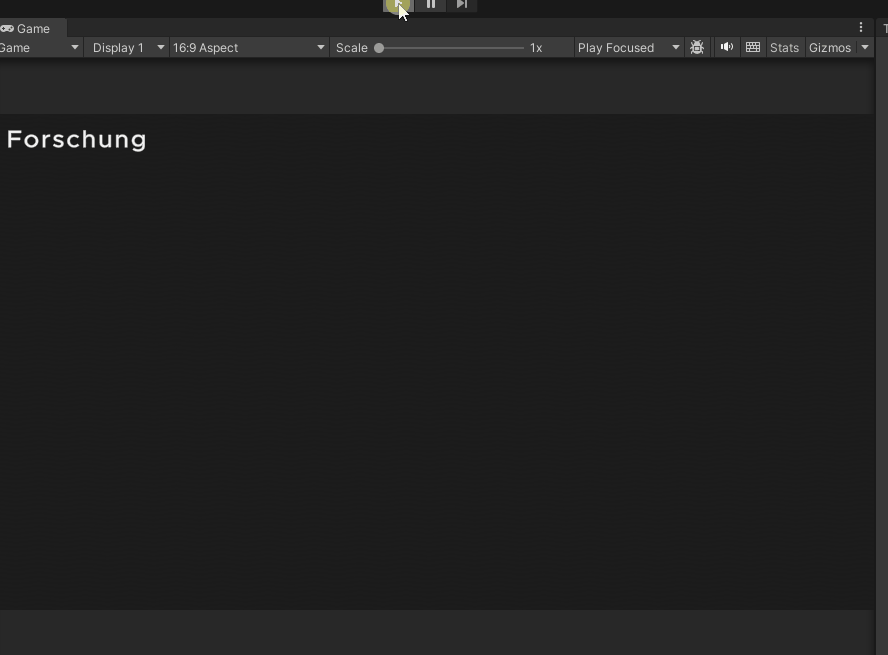
Nice. Let’s clean the code. „#region #endregion“ is a nice way to wrap code.
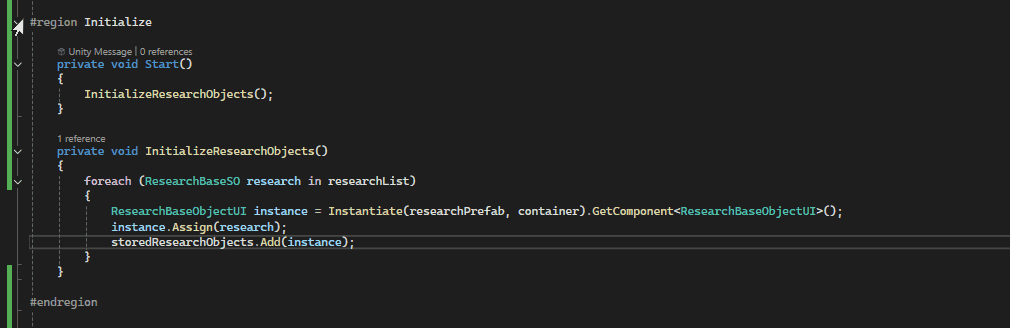