Façade Pattern in Unity (C# Design Pattern)
In Unity, it’s important to keep the Interface clean. Especially when we create larger projects. Thats why the Façade pattern is so useful. It hides the complexities of larger system.
For more Game Programming Patterns I can recommend: https://gameprogrammingpatterns.com/contents.html
#unity #design-pattern #programming #c-sharp #object-oriented-programming
What is the Façade Pattern?
The Façade Pattern is a structural design pattern that hides the complexities of larger system and provides a simpler interface.
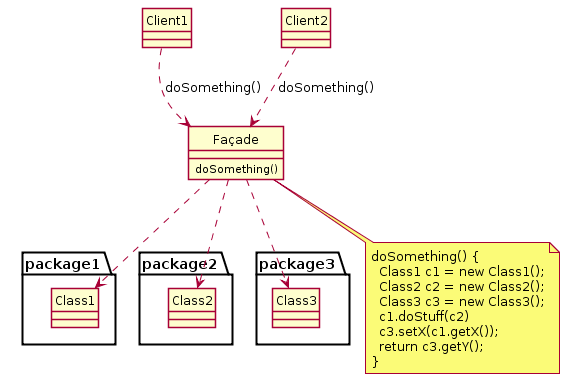
Subsystems
Using is only recommended when we already finished some classes that we can define as „Subsystems“.
Example standard classes:
- class PlayerManager – contains InitializePlayer() and ResetPlayer()
- class AudioManager – contains PlayBackgroundMusic() and StopBackgroundMusic()
- class LevelManager – contains LoadLevel(int level)
Code
short-form example:
public class PlayerManager
{
public void InitializePlayer() { Debug.Log("Player Initialized"); }
public void ResetPlayer() { Debug.Log("Player Reset"); }
}
public class AudioManager
{
public void PlayBackgroundMusic() { Debug.Log("Playing Background Music"); }
public void StopBackgroundMusic() { Debug.Log("Stopping Music"); }
}
public class LevelManager
{
public void LoadLevel(int level) { Debug.Log($"Loading Level {level}"); }
public void UnloadLevel(int level) { Debug.Log($"Unloading Level {level}"); }
}
The Façade Class
Create the class, in which we can control all Subsystems
public class GameFacade
{
private PlayerManager playerManager;
private AudioManager audioManager;
private LevelManager levelManager;
public GameFacade()
{
playerManager = new PlayerManager();
audioManager = new AudioManager();
levelManager = new LevelManager();
}
public void StartGame(int level)
{
levelManager.LoadLevel(level);
playerManager.InitializePlayer();
audioManager.PlayBackgroundMusic();
Debug.Log("Game Started!");
}
public void RestartGame(int level)
{
playerManager.ResetPlayer();
levelManager.UnloadLevel(level);
levelManager.LoadLevel(level);
audioManager.StopBackgroundMusic();
audioManager.PlayBackgroundMusic();
Debug.Log("Game Restarted!");
}
public void EndGame()
{
audioManager.StopBackgroundMusic();
Debug.Log("Game Ended!");
}
}
Done!
Now we can create the MonoBehaviour and be proud of our Façade !
public class GameController : MonoBehaviour
{
private GameFacade gameFacade;
void Start()
{
gameFacade = new GameFacade();
gameFacade.StartGame(1); // Start level 1
}
public void Restart()
{
gameFacade.RestartGame(1); // Restart level 1
}
public void EndGame()
{
gameFacade.EndGame(); // End the game
}
}