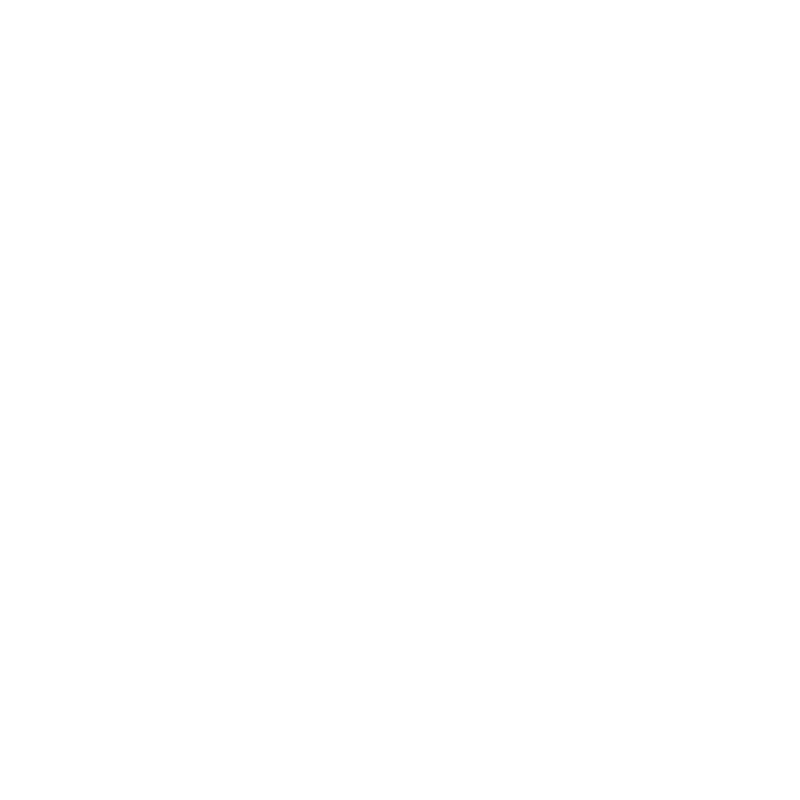
CPU vs GPU Comparison
Educational Project: Compute-Shader-Mining, SHA256-Hashing
What is a Hash-Algorithm? Basically: generating Millions of Hashes per Second→ searching for valid ones → repeat.
Tutorial: How to create a GPU & CPU Miner in Unity
In this Blog-Article we will create a Comparison between CPU vs. GPU hashing.
1. Create CPU- Miner
void Update()
{
float start = Time.realtimeSinceStartup;
for (int i = 0; i < hashesPerFrame; i++)
{
string input = baseInput + Random.Range(0, int.MaxValue);
string hash = Hash(input);
if (hash.StartsWith(targetPrefix))
{
Debug.Log("Valid Hash Found (CPU): " + hash);
}
}
float end = Time.realtimeSinceStartup;
currentHashrate = hashesPerFrame / (end - start);
}
string Hash(string input)
{
var bytes = Encoding.UTF8.GetBytes(input);
using var sha256 = SHA256.Create();
var hash = sha256.ComputeHash(bytes);
return System.BitConverter.ToString(hash).Replace("-", "").ToLower();
}
2. Create GPU- Miner
void Start()
{
resultBuffer = new ComputeBuffer(64, sizeof(uint));
}
void Update()
{
float start = Time.realtimeSinceStartup;
int kernel = computeShader.FindKernel("CSMain");
computeShader.SetBuffer(kernel, "results", resultBuffer);
computeShader.Dispatch(kernel, 1, 1, 1);
resultBuffer.GetData(results);
float end = Time.realtimeSinceStartup;
currentHashrate = results.Length / (end - start);
}
void OnDestroy()
{
resultBuffer.Release();
}
3. Create Shader
#pragma kernel CSMain
RWStructuredBuffer<uint> results;
[numthreads(64, 1, 1)]
void CSMain (uint3 id : SV_DispatchThreadID)
{
uint value = id.x + 12345;
value = (value ^ 0xABCDEF01) * 2654435761;
results[id.x] = value;
}
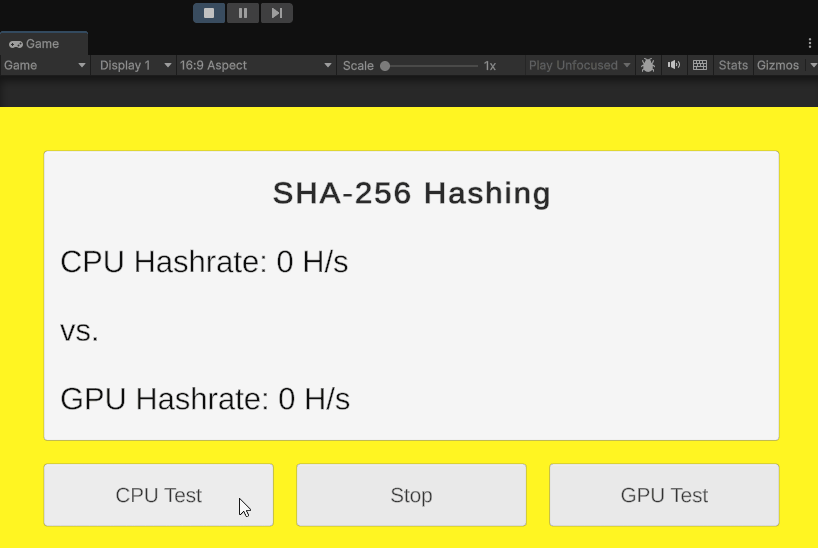
No responses yet